[기본 개념] 4 | (1.5) Math 클래스의 메서드
1 Object클래스
2 String클래스
3 StringBuffer클래스와 StringBuilder클래스
4> Math클래스
5 래퍼(wrapper)클래스
4. Math클래스
수학계산에 유용한 메서드로 구성되어 있다. Math클래스의 생성자는 접근제어자가 private이기 때문에 다른 클래스에서 Math인스턴스를 생성할 수 없도록 되어있다. 클래스 내에 인스턴스 변수가 하나도 없고, 메서드는 모두 static이며, 아래와 같이 2개의 상수만 정의해 놓았다.
Public static final double E = 2.7182818284590452354 ; // 자연로그의 밑
public static final double PI = 3.14159265358979323846 ; // 원주율
올림, 버림, 반올림
소수점 n번째 자리에서 반올림한 값을 얻기 위해서는 round( )를 사용해야 하는데, 항상 소수점 첫째 자리에서 반올림을 해서 정수 값(long)을 결과로 돌려준다.
사용자가 원하는 자리 수에서 반올림된 값을 얻기 위해서는 간단히 10의 n제곱으로 곱한 후, 다시 곱한 수로 나눠주기만 하면 된다.
1 원래 값에 100을 곱한다.
90.7552 * 100 --> 9075.52
2 위의 결과에 Math.round( )를 사용한다.
Math.round(9075.52) --> 9076
3 위의 결과를 다시 100.0으로 나눈다.
9076 / 100.0 --> 90.76
9076 / 100 --> 90
정수형 간의 연산에서는 반올림이 이뤄지지 않는다.
rint( )도 round( )처럼 소수점 첫째 자리에서 반올림하지만, 반환값이 double이다.
System.out.printf("round(%3.1f) = %d%n", 1.5, round(1.5)) // 반환값이 int
System.out.printf("rint(%3.1f) = %f%n", 1.5, rint(1.5)) // 반환값이 double
그리고 두 정수의 정가운데 있는 값은 가장 가까운 짝수 정수를 반환한다.
System.out.printf("round(%3.1f) = %d%n", -1.5, round(-1.5)) // -1
System.out.printf("rint(%3.1f) = %f%n", -1.5, round(-1.5)) // -1.0, -2.0의 정가운데이므로 -2.0 반환
예외를 발생시키는 메서드
메서드 이름에 'Exact'가 포함된 메서드들이 정수형 간의 연산에서 발생할 수 있는 오버플로우(overflow)를 감지하기 위해 추가되었다.
int addExact(int x, int y) // x + y
int subtractExact(int x, int y) // x - y
int multiplyExact(int x, int y) // x * y
int incrementExact(int a) // a++
int decrementExact(int a) // a--
int negateExact(int a) // -a
int toIntExact(long value) // (int) value - int로의 형변환
연산자는 단지 결과만 반환하지만 위의 메서드들은 오버플로우가 발생하면, 예외(ArithmeticException)를 발생시킨다.
삼각함수와 지수, 로그
두 점 (x1, y2), (x2, y2)간의 거리 c는
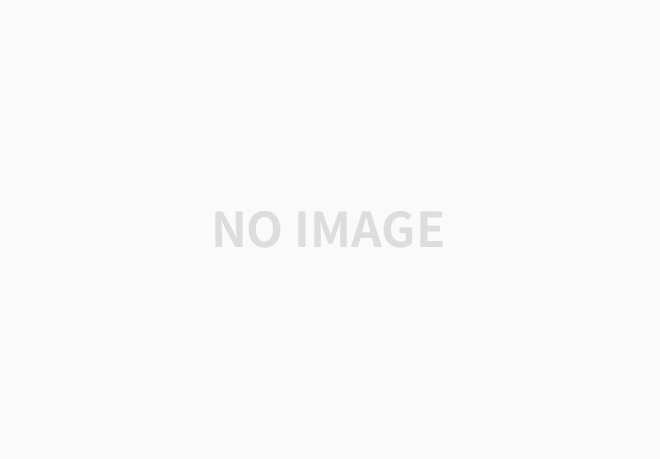
로 구할 수 있다.
제곱근을 계산해주는 sqrt( )와 n제곱을 계산해주는 pow( )를 사용해서 식을 구성하면 다음과 같다.
(x1, y1)를 (1, 1), (x2. y2)를 (2, 2)라고 하자.
double c = sqrt(pow(x2 - x1, 2) + pow(y2 - y1, 2)) ;
--> double c = sqrt(pow(2 - 1, 2) + pow(2 - 1, 2)) ;
--> double c = sqrt(pow(1, 2) + pow(1, 2)) ; // pow(1, 2)는 1의 2제곱
--> double c = sqrt(1.0 + 1.0) ;
--> double c = sqrt(2.0) ;
--> double c = 1.414214 ;
이외에도 각도를 라디안으로 변환하는 toRadians( ), 라디안을 각도로 변환하는 toDegrees( )등의 메서드가 있다.
StrictMath클래스
예를 들어, 부동소수점 계산의 경우 반올림의 처리방법 설정이 OS마다 다를 수 도 있기 때문에 결과가 다를 수도 있는데 이러한 차이를 없애기 위해 항상 같은 결과를 얻도록 Math클래스를 새로 작성한 클래스이다.
Math클래스의 메서드
메서드 / 설명 | 예제 | 결과 |
static double abs(double a) static float abs(float f) static int abs(int f) static long abs(long f) |
int i = Math.abs(-10) ; double d = Math.abs(-10.0) ; |
i = 10 d =10.0 |
주어진 값의 절대값을 반환한다. | ||
static double ceil(double a) | double d = Math.ceil(10.1) ; double d2 = Math.ceil(-10.1) ; double d3 = Math.ceil(10.000015) ; |
d = 11.0 d2 = -10.0 d3 = 11.0 |
주어진 값을 버림하여 반환한다. | ||
static double floor(double a) | double d = Math.floor(10.8) ; int i = Math.floor(-10.8) ; |
d = 10.0 d2 = -11.0 |
주어진 값을 버림하여 반환한다. | ||
static double max(double a, double b) static float max(float a, float b) static int max(int a, int b) static long max(long a, long b) |
double d = Math.max(9.5, 9.50001) ; int i = Math.max(0, -1) ; |
d = 9.50001 i = 0 |
주어진 두 값을 비교하여 큰 쪽을 반환한다. | ||
static double min(double a, double b) static float min(float a, float b) static int min(int a, int b) static long min(long a, long b) |
double d = Math.min(9.5, 9.50001) ; int i = Math.min(0, -1) ; |
d = 9.5 i = -1 |
주어진 두 값을 비교하여 작은 쪽을 반환한다. | ||
static double random( ) | double d = Math.random( ) ; int i = (int)(Math.random( ) * 10) + 1 ; |
0.0 <= d < 1.0 1 <= i < 11 |
0.0 ~ 1.0범위의 임의의 double값을 반환한다. | ||
static double rint(double a) | double d = Math.rint(1.2) ; double d2 = Math.rint(2.6) ; double d3 = Math.rint(3.5) ; double d4 = Math.rint(4.5) ; |
d = 1.0 d2 = 3.0 d3 = 4.0 d4 = 4.0 |
주어진 double값과 가장 가까운 정수값을 double형으로 반환한다. 단, 두 정수의 정가운데 있는 값(1.5, 2.5, 3.5)은 짝수를 반환한다. |
||
static long round(double a) static long round(float a) |
long l = Math.round(1.2) ; long l2 = Math.round(2.6) ; long l3 = Math.round(3.5) ; long l4 = Math.round(4.5) ; double d = 90.7552 ; double d2 = Math.round(d * 100) / 100.0 ; |
l = 1 l2 = 3 l3 = -4 l4 = -5 d = 90.7552 d2 = 90.76 |
소수점 첫째자리에서 반올림한 정수값(long)을 반환한다. 매개변수의 값이 음수인 경우, round( )와 rint( )의 결과가 다르다는 것에 주의하자. |
출처 | Java의 정석 (남궁 성)
'💠프로그래밍 언어 > Java' 카테고리의 다른 글
[기본 개념] 4 | (2.1) Java.util.Object 클래스, Java.util.Random 클래스 (0) | 2021.11.29 |
---|---|
[기본 개념] 4 | (1.6) Wrapper 클래스 (1) | 2021.11.29 |
[기본 개념] 4 | (1.4) StringBuffer클래스, StringBuilder 클래스의 메서드 (0) | 2021.11.26 |
[기본 개념] 4 | (1.3) String 클래스의 메서드 (0) | 2021.11.26 |
[기본 개념] 4 | (1.2) Object 클래스의 메서드 (0) | 2021.11.26 |